Looping statements or loops in C/C++ are the special kinds of statements which when encountered by the control of execution, execute the next statement or the block of statements repeatedly.
Components of a Loop Statement
Following are the main parts or components of a loop in C/C++:
- Loop Variable/Counter: This is a variable defined before the loop statement or within the loop statement. It controls and counts the number of times the loop body will be executed. The value of the loop counter is updated after each cycle or execution of the loop statement.
- Initialization Expression: This initializes the loop variable with an initial or starting value. It is executed only once i.e. during the very first cycle of the loop.
- Test/Conditional Expression: This is the most crucial component of any loop statement because it checks the defined condition for the execution of the loop body. It is executed every time (in each cycle) before entering into the loop body.
- Update Expression: It is the other most important component of the loop statement which updates the value of the loop counter/variable and controls the number of iterations i.e. the frequency of execution of the loop body.
- Loop Body: This is a statement or a group/block of statements that get executed depending upon the truth value of the test statement.
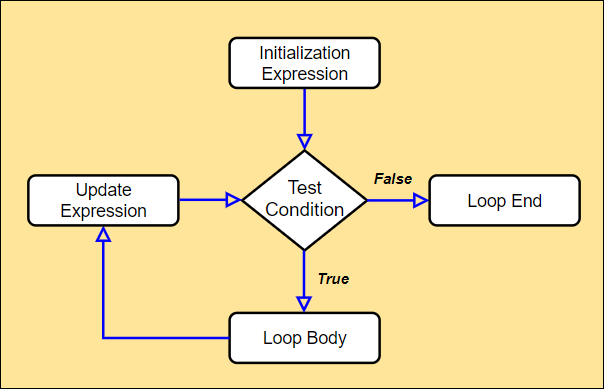
In this tutorial, we are going to discuss the following pointers regarding the looping statements in C/C++.
- Types of Loops
- for loop
- while loop
- do while loop
- nested loops
Types of Loops
In C/C++ there are mainly two types of looping statements or loops.
1. Entry Controlled Loops
The kind of loops that checks the looping or test condition before the execution of the loop body (a single statement or a block of statements).
It does not execute the loop body if the loop/test condition is false.
Examples: for loop and while loop
2. Exit Controlled Loops
The kind of loops that checks the looping or test condition after executing the loop body (a single statement or a block of statements).
It executes the loop body at least once even if the loop/test condition is false.
It is used to make menu driven program.
Example: do while loop
for loop
It is one of the most widely used looping statements in C/C++.
Syntax:
for (initialization expression; test expression; update expression)
{
statements;
// loop body
}
NOTE: for loop is used when the number of iterations i.e. the number of times the loop will be executed is known to us.
C++ program using for loop:
// C++ program to print the table of five
#include <iostream>
using namespace std;
int main()
{
for (int i = 1; i <= 10; i++)
cout<<"5 "<<"x "<<i<<" = "<<5*i<<endl;
return 0;
}
Output:
Table of five (5):
5 x 1 = 5
5 x 2 = 10
5 x 3 = 15
5 x 4 = 20
5 x 5 = 25
5 x 6 = 30
5 x 7 = 35
5 x 8 = 40
5 x 9 = 45
5 x 10 = 50
while loop
An another most widely used looping statement in C/C++.
Syntax:
initialization expression;
while (test expression)
{
statements;
// loop body
update expression;
}
NOTE: while loop is used when the number of iterations is not known to us.
C++ program using while loop:
// C++ program to print the first five even Numbers
#include <iostream>
using namespace std;
int main()
{
int i = 2, count = 5;
cout<<"First five even Nos are:"<<endl;
while (count > 0)
{
cout<<i<<" ";
count--;
i = i +2;
}
return 0;
}
Output:
First five even Nos are:
2 4 6 8 10
Also read Five Ways to Calculate Power in C++
do while loop
It is also an important looping statement but it is used in very limited cases.
Syntax:
initialization expression;
do
{
statements;
// loop body
update expression;
} while (test expression);
NOTE: do while loop is used when we need the execution of the loop body at least once even if the test condition is false.
C++ program using do while loop:
// C++ program program to print a string five times
#include <iostream>
using namespace std;
int main()
{
int i = 0;
do
{
cout<<"This is a do while loop!"<<endl;
i++;
} while (i != 5);
return 0;
}
Output:
This is a do while loop!
This is a do while loop!
This is a do while loop!
This is a do while loop!
This is a do while loop!
Nested loops
This is an advanced and very useful use of looping statements, in which one loop contains one or more loops in its loop body
initialization expression;
while (test expression)
{
statements;
for (initialization expression; test expression; update expression)
{
statements;
// loop body
}
// loop body
update expression;
}
C++ program using nested loops:
// C++ program to print the tables of the first five numbers
#include <iostream>
using namespace std;
int main()
{
int k = 1;
cout<<"Tables of first five numbers:"<<endl;
while(k <= 5)
{
for (int i = 1; i <= 10; i++)
cout<<k * i<<" ";
cout<<endl;
k++;
}
return 0;
}
Output:
Tables of first five numbers:
1 2 3 4 5 6 7 8 9 10
2 4 6 8 10 12 14 16 18 20
3 6 9 12 15 18 21 24 27 30
4 8 12 16 20 24 28 32 36 40
5 10 15 20 25 30 35 40 45 50